魔镜开发日记(一)软件模拟串口通信--SoftwareSerial库的使用
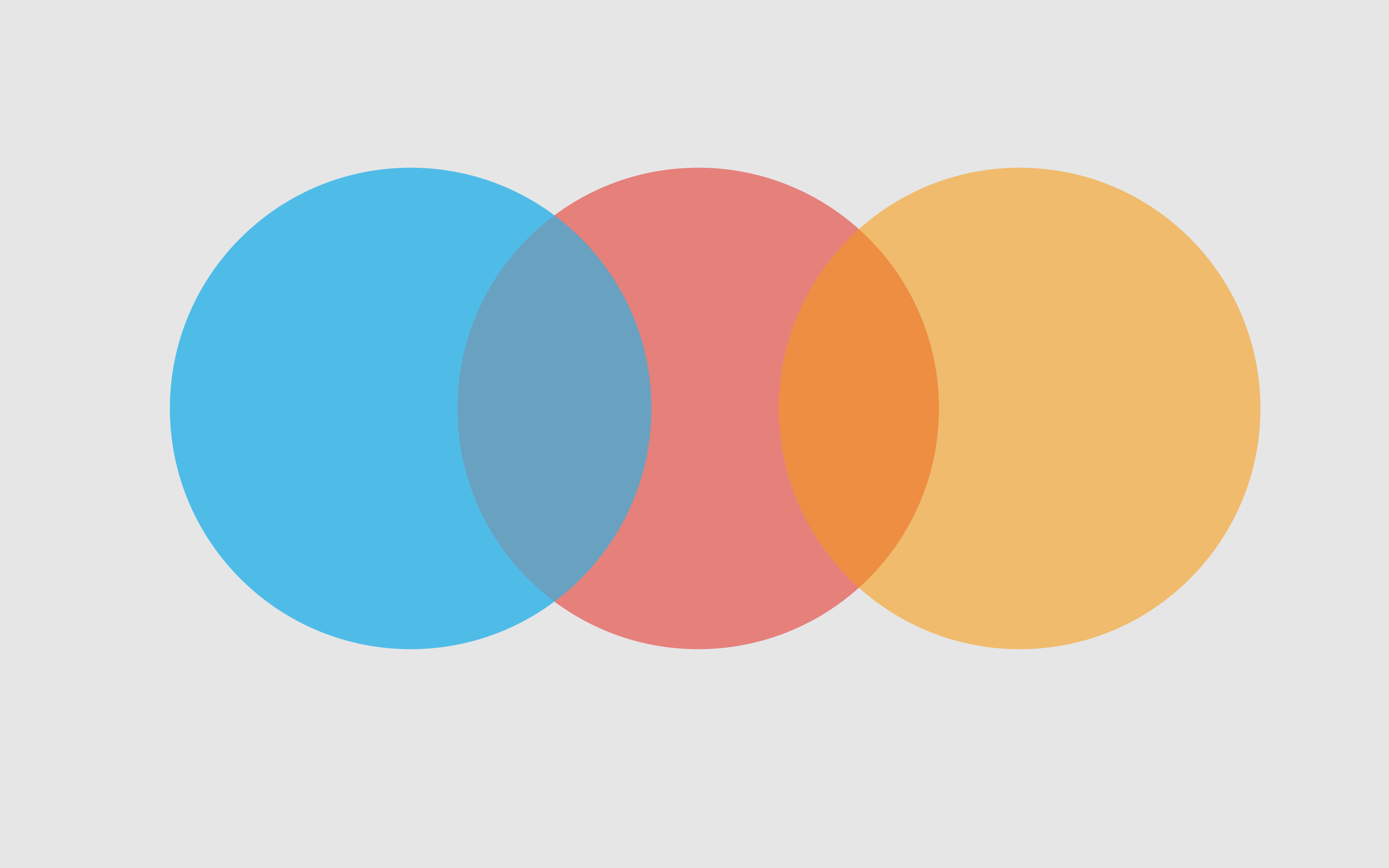
魔镜开发日记(一)软件模拟串口通信--SoftwareSerial库的使用
余生项目涉及:Arduino与蓝牙模块通信
通常我们将Arduino UNO上自带的串口称为硬件串口,而使用SoftwareSerial类库模拟成的串口,称为软件模拟串口(简称软串口)。
- 硬件串口:0,1引脚,分别为RX,TX
软串口接收引脚波特率建议不要超过57600
SoftwareSerial类成员函数
该类库不在Arduino核心类库中,需要进行声明
SoftwareSeial()
- 作用:通过它指定软串口的RX,TX引脚
- 语法:SoftwareSerial mySerial= SoftwareSerial(rxPin, txPin)
SoftwareSerial mySerial(rxPin, txPin) - 参数:myserial:用户自定义软件串口对象
rxPin:软串口接收引脚
txPin:软串口发送引脚
listen()
- 作用:开启软串口监听状态
Arduino在同一时间仅能监听一个软串口 - 语法:myserial.listen()
isListening()
- 作用:监测软串口是否正在监听状态。
- 语法:mySerial.isListening()
- 参数:
mySerial:用户自定义的软件串口对象 - 返回值:
Boolean型
True:正在监听
False:没有监听
end()
- 作用:停止监听软串口。
- 语法:mySerial. end()
- 参数:
mySerial:用户自定义的软件串口对象 - 返回值:
Boolean型
True:关闭监听成功
False:关闭监听失败
overflow()
- 作用:检测缓冲区是否溢出。
- 语法:mySerial.overflow()
- 参数:mySerial:用户自定义的软件串口对象
- 返回值:
Boolean型
True:溢出
False:没有溢出
示例代码
1 | /* |